The Last Ride Together
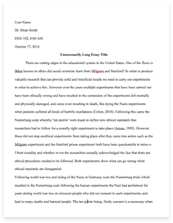
- Pages: 3
- Word count: 608
- Category: Mathematics
A limited time offer! Get a custom sample essay written according to your requirements urgent 3h delivery guaranteed
Order NowQuestion 1:
Write a program to input a start limit S (S>0) and the last limit L (L>0). Print all the prime triplets between S and L (both inclusive), if S<=L otherwise your program should ask to re-enter the values of S and L again with a suitable error message.
Algorithm:
* Start
* To input the lower limit
* To input the upper limit
* To run the outer loop
* To run inner loop
* To calculate total number of prime numbers between lower and upper limits
* To declare array with it’s number of elements as ‘s’
* To run the outer loop
* To run the inner loop
* To store the prime numbers in array a[]
* To run a loop for every position of array a[]
* If condition matches for the number for prime triplets
* Continue till all Prime Triplets are printed
Question 2:
A unique digit integer is a positive integer (without leading zeros) with no duplicate digits. For example 7, 135, 214 are all unique digit integers whereas 33, 3121, 300 are not. Given two positive integers m and n, where m<n, write a program to determine how many unique digit integers are there in the range between m and n (both inclusive) and output them.
Algorithm:
* Start
* To input the starting limit
* To input the last limit
* To run the outer loop
* To store the value of ‘i’ as a string
* To run the inner loop
* To run a nested loop of the inner loop
* To check for repetition of any digit in the number
* To store all the unique digit integers in a string
* To store the frequency of unique digit integers
* To print the unique digit integers and their frequency
Question 3:
Write a program which inputs Natural numbers N and M followed by integer arrays A[ ] and B[ ], each consisting of N and M numbers of elements respectively. Sort the arrays A[ ] and B[ ] in Ascending order of magnitude. Use the sorted arrays A[ ] and B[ ] to generate a merged array C[ ] such that the elements of A[ ] and B[ ] appears in C[ ] in Ascending order without any duplicate elements. Sorting of array C[ ] is not allowed.
Algorithm:
* Start
* To enter the limit of first array (<21)
* To enter the limit of the second array (<21)
* To run a loop
* To enter the two arrays
* To sort the two arrays using bubble sort technique
* To copy the two arrays into a single array
* To sort the merged array using bubble sort technique
* To run a loop
* To print the elements of the i-th position
* To go to the next index of the next number
Question 4:
Write a program to input and store n integers (n > 0) in a single subscripted variable and print each number with their frequencies of existence. The output should contain number, asterisk symbol and its frequency and be displayed in separate lines.
Algorithm:
* Start
* To enter capacity
* To enter the numbers in an array
* To run outer loop
* To run inner loop
* To sort the numbers in the array
* To run outer loop
* To run inner loop
* To transfer the values of the array in another array
* To check frequency and print
Question 5:
Write a program to input an arithmetic expression in String form which contains only one operator between two numeric operands. Print the output in the form of number. ( If more than one operators are present, an error output message “ INVALID EXPRESSION” should appear).